Tutorial: Calculating the Pi Top Cycle Indicator
November 24, 2024
This tutorial walks you through the steps to use TAAPI.IO’s Bulk Query feature to calculate the Pi Top Cycle Indicator, which utilizes two SMA (Simple Moving Average) values. We’ll be calculating it for Bitcoin (BTC/USDT).
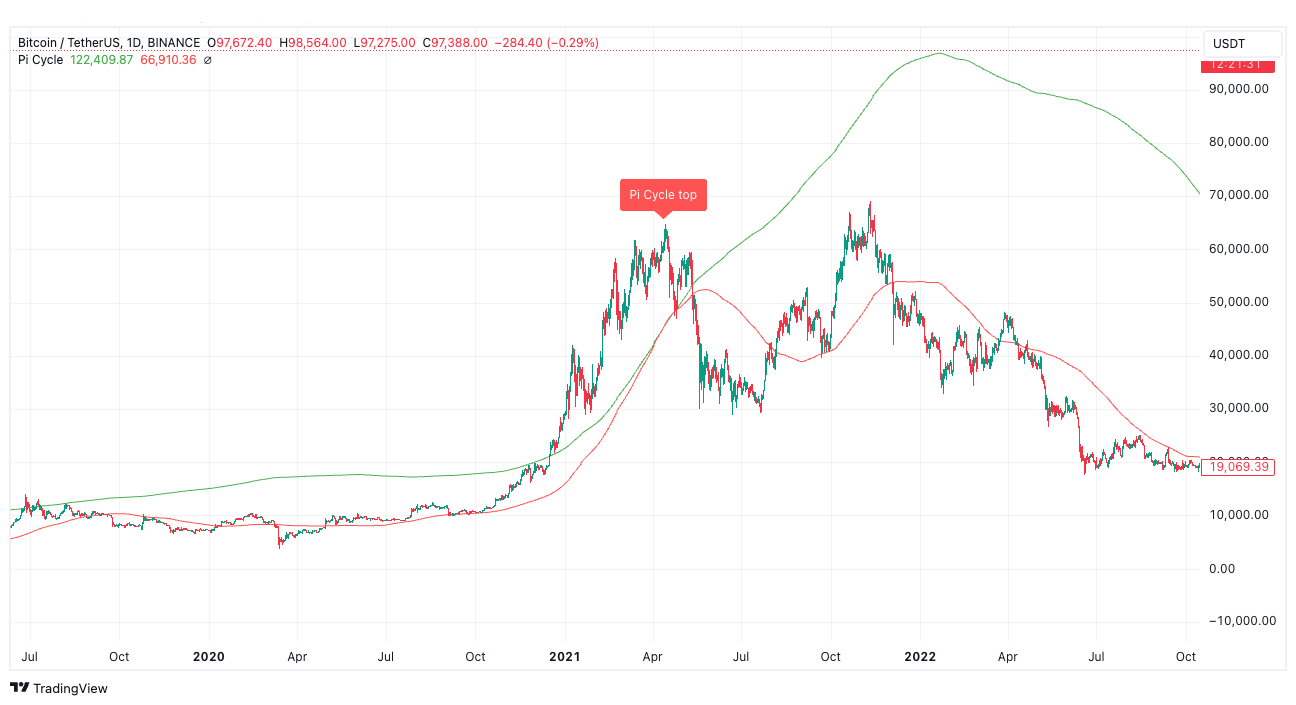
What Is the Pi Top Cycle Indicator?
The Pi Top Cycle Indicator is a long-term market indicator used to identify market cycle tops in cryptocurrencies. It is calculated using:
- 350-day SMA multiplied by 2.
- 111-day SMA.
By comparing these two values, traders can assess potential cycle peaks.
Step 1: Prerequisites
- TAAPI.IO Account: Ensure you have an account on TAAPI.IO and obtain your API key (referred to as secret in this tutorial).
- API Tool: Use any tool to make API requests, such as Postman, Curl, or a coding language like Python or JavaScript.
Step 2: Understanding the Bulk Query
The Bulk Query allows you to fetch multiple indicators in a single request. Here’s the query we will use:
{
"secret": "YOUR_TAAPI_IO_SECRET",
"construct": {
"exchange": "binance",
"symbol": "BTC/USDT",
"interval": "1d",
"indicators": [
{
"id": "350_DMA_multiply*2",
"indicator": "sma",
"period": 350
},
{
"id": "111_DMA",
"indicator": "sma",
"period": 111
}
]
}
}
Step 3: Sending the Request
- Replace YOUR_TAAPI_IO_SECRET with your actual API key.
- Send the request to the TAAPI.IO Bulk Endpoint:
POST https://api.taapi.io/bulk
- For example, using Curl:
curl -X POST https://api.taapi.io/bulk \
-H "Content-Type: application/json" \
-d '{
"secret": "YOUR_TAAPI_IO_SECRET",
"construct": {
"exchange": "binance",
"symbol": "BTC/USDT",
"interval": "1d",
"indicators": [
{
"id": "350_DMA_multiply*2",
"indicator": "sma",
"period": 350
},
{
"id": "111_DMA",
"indicator": "sma",
"period": 111
}
]
}
}'
Step 4: Processing the Response to get the Pi Top Cycle
After sending the request, you’ll receive a response with the SMA values:
{
"350_DMA_multiply*2": {
"value": 60000
},
"111_DMA": {
"value": 58000
}
}
1. Extract the 350-day SMA value and multiply it by 2.
• Example: 350_DMA_multiply*2 = 60000
2. Compare it with the 111-day SMA value.
• Example: 111_DMA = 58000
Step 5: Interpret the Pi Top Cycle
• If the 111-day SMA approaches or exceeds the 2 × 350-day SMA, it may indicate a market cycle top.
• Conversely, if the 111-day SMA is significantly below, the market is likely far from a cycle top.
Step 6: Automating with Code
You can automate this process in Python:
import requests
# TAAPI.IO secret key
API_SECRET = "YOUR_TAAPI_IO_SECRET"
# Define the bulk request
payload = {
"secret": API_SECRET,
"construct": {
"exchange": "binance",
"symbol": "BTC/USDT",
"interval": "1d",
"indicators": [
{"id": "350_DMA_multiply*2", "indicator": "sma", "period": 350},
{"id": "111_DMA", "indicator": "sma", "period": 111},
],
},
}
# Send the request
response = requests.post("https://api.taapi.io/bulk", json=payload)
data = response.json()
# Extract and calculate
sma_350_multiplied = data["350_DMA_multiply*2"]["value"]
sma_111 = data["111_DMA"]["value"]
print(f"350 DMA * 2: {sma_350_multiplied}")
print(f"111 DMA: {sma_111}")
if sma_111 >= sma_350_multiplied:
print("Potential market cycle top!")
else:
print("Market is below the cycle top.")
Conclusion
Using TAAPI.IO’s Bulk Query simplifies fetching multiple indicator values, making it ideal for calculating and using the Pi Top Cycle Indicator. Automating this process can save time and help in quick decision-making during volatile market conditions.