Building on the previous guide Strategies – Framework [basics], we will set up a fully functional multi strategies setup. If you haven’t already read this guide, please do so to learn the basics.
This guide is not meant to be advanced, but basic coding knowledge is assumed. Additionally, this setup is cross-platform, so you will be able to run this on any platform [Linux, Mac, Windows, etc…], and basic Linux Bash commands are used in these examples on the command line, but do things how you feel comfortable when creating files and folders on your platform.
Prerequisites
- Read the Strategies – Framework [basics] guide
- Basic understanding of Javascript coding
- Git installed
- Docker installed
- Docker Compose installed
Note that, we don’t even need to have NodeJS nor MongoDB installed, as Docker takes care of this automatically. But it’s a good idea to have NodeJS installed while developing so that your IDE can help out.
Getting started
To get started we first have to “Clone” the TAAPI.IO Strategies repository where we can then change the remote to point to our private account. Steps are simple:
- Clone: https://github.com/taapi/strategies
- Change directory to: strategies
- Change Git remote
- Push
Then we’ll need a folder to put our project in:
> cd my-projects
> git clone https://github.com/taapi/strategies
> cd strategies
> git remote set-url origin https://github.com/your-username/private-repo.git
> git push -u origin main
# If you have NodeJS installed
> cd strategy
> npm install # Install all dependencies
Be sure to replace “your-username” with your own actual username, along with actual private repository!
You should now have a complete copy of TAAPI.IOs open source strategies repository, along with all the strategies we’ve created for public use.
Boot up your favorite code IDE and verify that you see something similar to the below directory / files structure.
> code .
Directory / files Structure
taapi-strategies/
|-- strategies/
| |-- phobos
| | |-- README.md
| | |-- Scanner.js
| | |-- State_<your-state-name>.js
| | |-- State_start.js
| | |-- State.js
| | |-- strategy.yml
| |-- <other strategies>
|
|-- strategy/
| |-- node_modules
| |-- src/
| | |-- index.js
| |
| |-- config.yml # Missing at clone time
| |-- package-lock.json
| |-- package.json
|
|-- .env # Missing at clone time
|-- .gitignore
|-- docker-compose.yml
Lets have a look at the different files.
In our taapi-strategies/strategies/phobos
folder, we see 6 files specifically for the phobos
bot:
- README.md – A Markdown description of the bot
- Scanner.js # Implementation of this bots scanner
- State.js # All state implementations should inherit from this file (or extend it)
- State_start.js # A mandatory “start state” implementation
- State_long.js # Long implementation
- State_short.js # Short implementation
- strategy.yml – Configuration for this unique strategy
In the taapi-strategies/strategy
folder we also see 6 items:
- node_modules # Contains all app dependencies needed to run the app (if you installed dependencies)
- src/index.js # Main entry point. This is the file that boots up the framework
- config.yml # Global configuration file. All strategy implementations will inherit this config
- package-lock.json # NodeJS package lock file tracking the installed versions of all dependencies
- package.json # NodeJS package file holding runtime and dependency config
Lastly, 3 files in the taapi-strategies
root folder:
- .env # Environment file, instructing Docker which ports and strategy code to boot up
- .gitignore # A list of files and folders to be ignored by Git. Usually sensitive information is ignored, like configurations etc…
- docker-compose.yml # The Docker Compose configuration, which instructs Docker how to boot up
The only files missing are the strategy/src/config.yml
, and .env
. Please create those files, and fill in the below configuration.
Note: Be sure to paste in your TAAPI.IO Secret! If you don’t have one, then get one.
server:
env: "dev"
host: "localhost"
port: 3002
debugMode: true
api:
corsOrigin: "*"
user: "user"
pass: "pass"
database:
namePrefix: "taapi_strategies_"
name: "${BOT_ID}" # Will be replaced with the actual bot id in the strategies.yml file at run time!
host: "mongo"
port: 27017
user: ""
pass: ""
protocol: "mongodb"
collectionPrefix: ""
replSetName: null
sslValidate: false
sslCertFilename: null
exchange:
id: "whitebitcollateral"
name: "Whitebit"
logging:
errors: true
stateChanges: true
ta: false
uptimerobot:
heartbeatUrl: ""
taapi:
secret: ""
notifications:
slack:
webhookUrl: null
channel: "bot"
username: "TAAPI.IO Strategies"
icon: "information_source"
telegram:
botKey: null
chatId: null
bot_port=3002
app_version="dev"
strategy_id="phobos"
And this is it for the setup. We’re now ready to start running things.
How it works
Now, for beginners that haven’t worked much with Docker or Docker Compose, this might seem like a lot of magic. But unless you have an urge to spend a lot of hours of your life understanding how and why this works, don’t worry about it.
But essentially what happens behind the scenes is that docker-compose copies the source code for the strategy defined in the .env file. So in this case with the strategy set to phobos
, Docker Compose will copy everything in taapi-strategies/strategies/phobos
into the runtime container, and boot up this code to run on port 3002
.
All we have to do now, is run one command:
> docker-compose up
If things are working how they should, you should see something like this
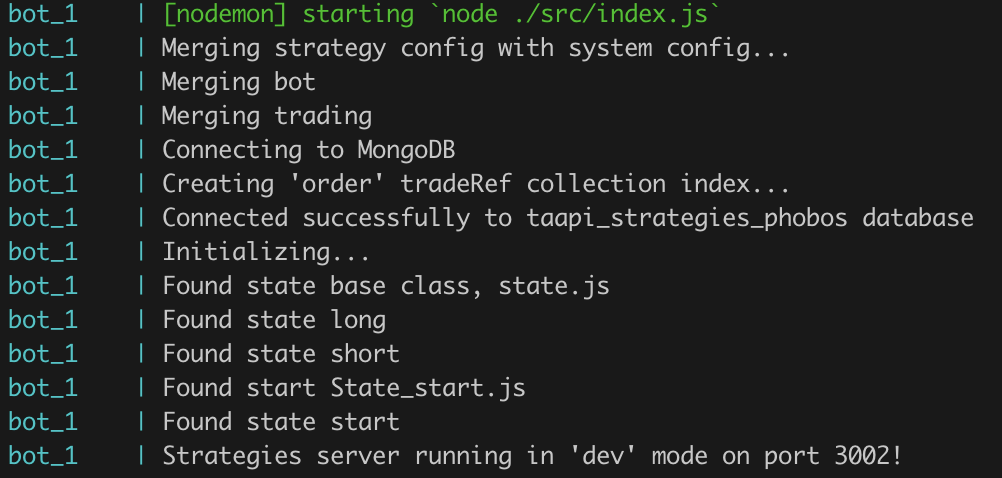
So what happens in the background, is that first, Docker will look at your system and check if all dependencies are installed. This means a virtual Linux Operating System, NodeJS, MongoDB, and all program packages needed to run the APP.
This might take a minute or two the first time, but once it’s done, you should see something similar to the above output. Don’t worry, all of these commands are safe to run. No additional software will be installed on your system, everything is running in so-called “containers” which is basically a very tiny Virtual Machine running on your host system.
Changing strategy
Say that your implementation of the bot Phobos
is complete, and you’re focussing your attention to a new bot Deimos
, the second moon of Mars. Simply shut down your current bot by hitting ctrl-c
, change the strategy_id
in the .env
file, and run docker-compose up
again.
The folder name for the bot in taapi-strategies/strategies/
is an exact match for the strategy_id
in the configuration files, so be sure to name those precisely.
bot_port=3002
app_version="dev"
strategy_id="deimos"
Development
Now we’re up and running and we can now start focusing on what this is all about – the strategies.